Welcome to the Atlas Device SDK Docs
Atlas Device SDK is a suite of app development tools optimized for data access and persistence on mobile and edge devices. Use the SDKs to build data-driven mobile, edge, web, desktop, and IoT apps.
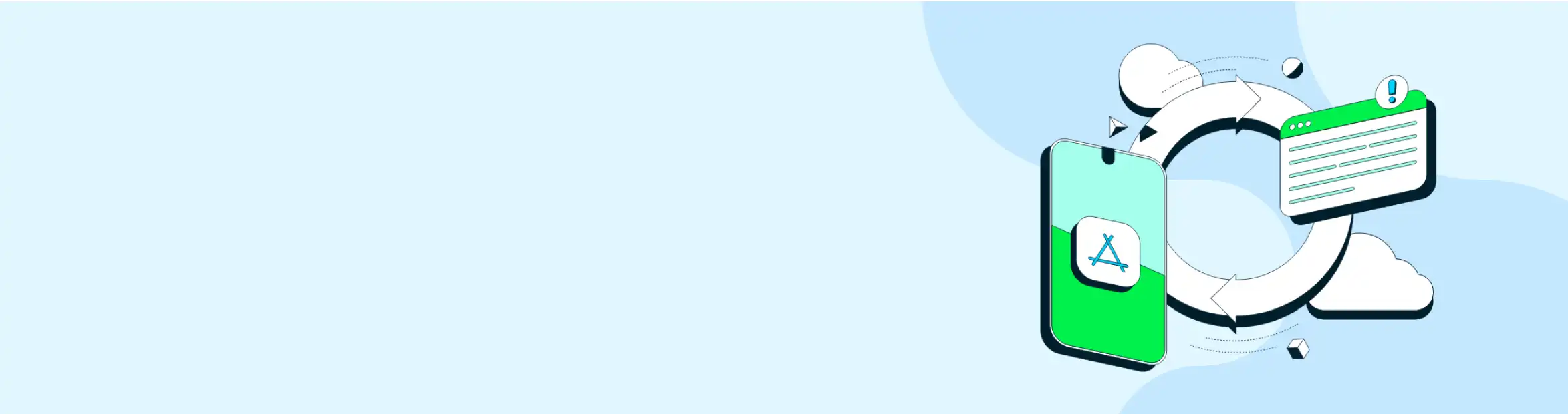
The SDKs provide tools to read and write Atlas data from devices. Your app can sync automatically with MongoDB Atlas and other devices using Device Sync. You can call Atlas Functions from a device. The device persistence layer is Realm, an embedded, object-oriented database that lets you build real-time, offline-first applications.
We provide SDKs for most popular languages, frameworks, and platforms. Each SDK is language-idiomatic and includes:
The core database APIs for creating and working with on-device databases.
The APIs you need for connecting to the App Services backend so you can make use of server-side features like Device Sync, Authentication, Functions, Triggers, and more.
Get Started with Atlas Device SDK
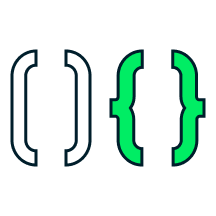
Quick Start
Minimal-explanation code examples of how to work with the Swift SDK. Write to the device database, and sync with other devices.
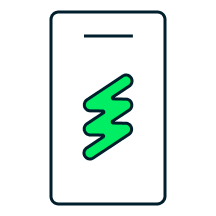
Working Example App
Learn from example by dissecting a working mobile client app that uses Atlas Device SDK.
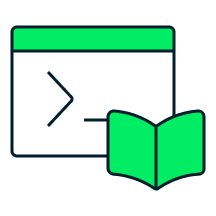
Guided Tutorial
Follow a guided tutorial to learn how to adapt the example app to create your own working app.
Develop Apps with the SDK
Use the SDK's open-source database - Realm - as an object store on the device. Use Device Sync to keep data in sync with your MongoDB Atlas cluster and other clients.
Install the SDK
Use popular package managers and build tools to Install Atlas Device SDK in your project.
Import the relevant dependency in your project files to get started.
Define an Object Schema
Use your preferred programming language to idiomatically define an object model.
Open a Database
The SDK's database - Realm - stores objects in files on your device. Or you can open an in-memory database which does not create a file. Configure and open a database to specify the options for your database file.
Read and Write Data
Create, read, update, and delete objects from the device database. Filter data using the query engines provided by the SDK.
React to Changes
Live objects mean that your data is always up-to-date. You can register a notification handler to watch for changes and perform some logic, such as updating your UI. Or use our React Native or SwiftUI support to idiomatically to update Views when data changes.
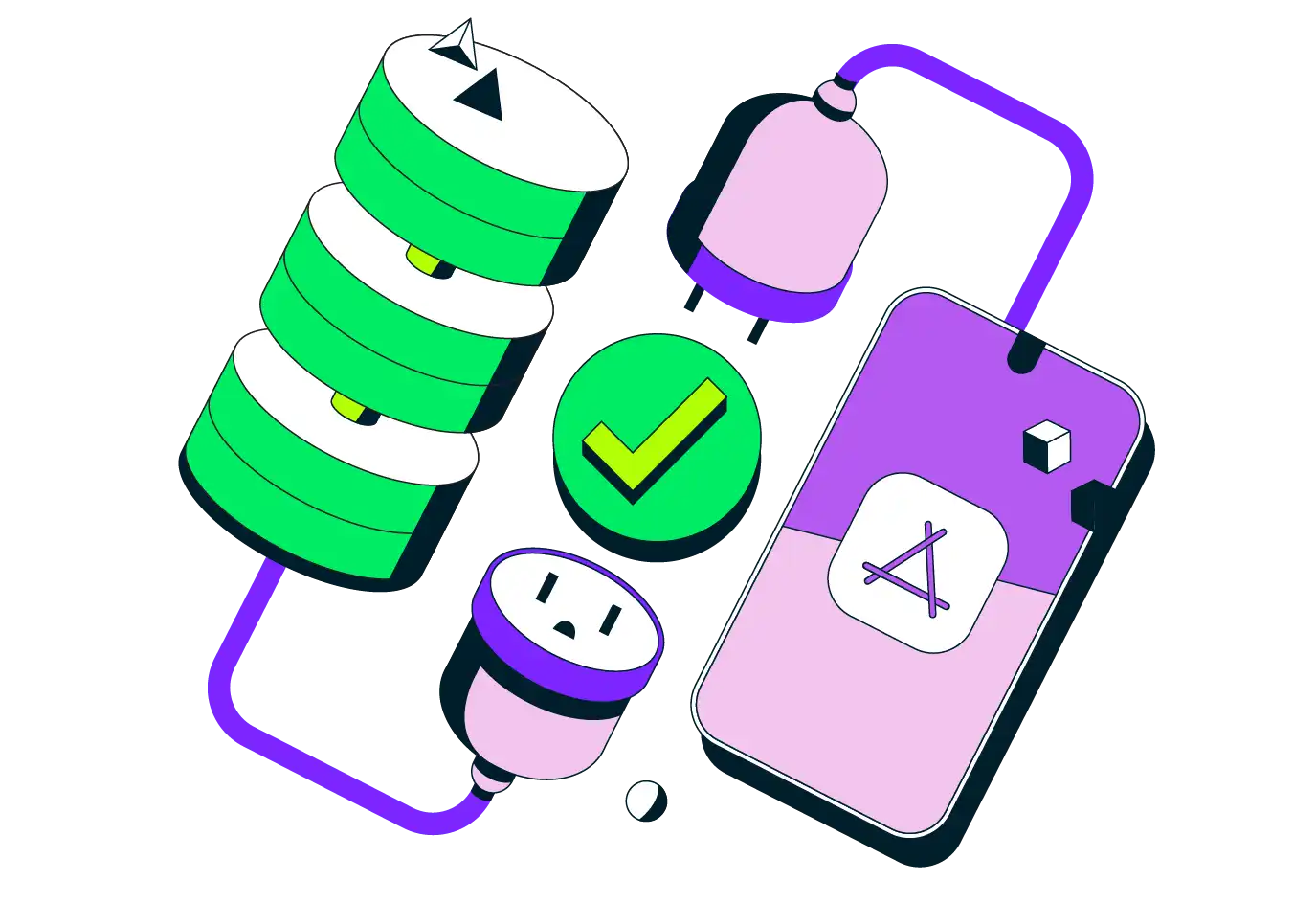
Connect to an Atlas App Services App
Configure Device Sync in an App Services App. Define data access rules. Use Development Mode to infer your schema from your client data model.
Then, connect to Atlas from your client.
Authenticate a User
Use one of our authentication providers to authenticate a user. App Services provides access to popular authentication providers, such as Apple, Google, or Facebook. Use our built-in email/password provider to manage users without a third-party, or use custom JWT authentication to integrate with other authentication providers. Anonymous authentication provides access without requiring a login or persisting user data.
Open a Synced Database
Configure and open a synced database. Subscribe to a query to determine what data the synced database can read and write.
Read and Write Synced Data
The APIs to read and write data are the same whether you're using a synced or non-synced database. Data that you read and write to the device is automatically kept in sync with your Atlas cluster and other clients. Apps keep working offline and deterministically sync changes whenever a network connection is available.
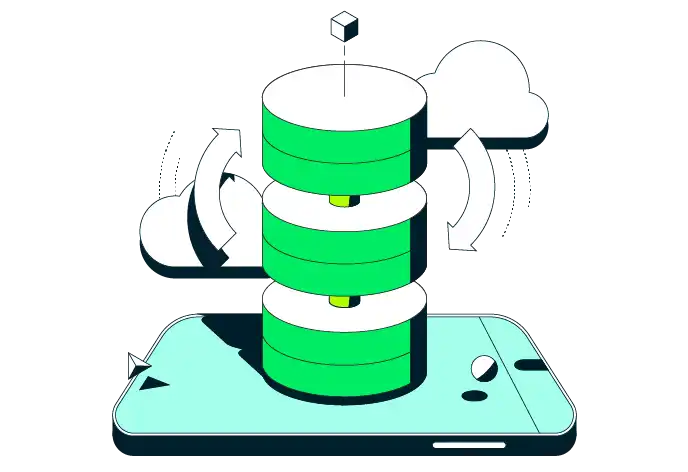
Call Serverless Functions
You can call serverless Functions from your client application that run in an App Services backend.
Query MongoDB Atlas
You can query data stored in MongoDB directly from your client application code.
Authenticate Users
Authenticate users with built-in and third-party authentication providers. Use the authenticated user to access App Services.
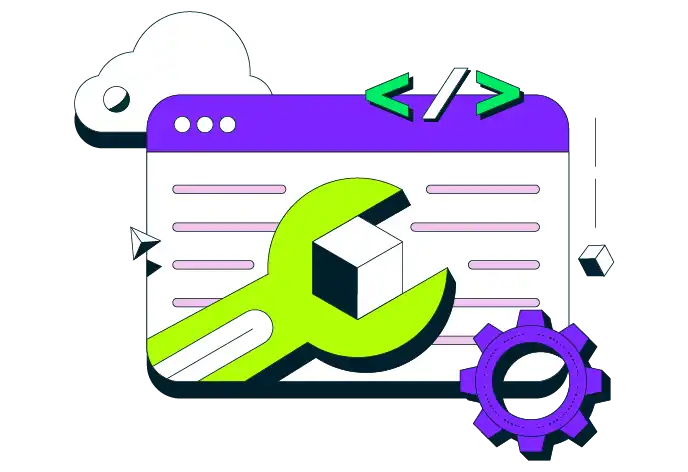
Our SDKs offer framework-idiomatic tools to streamline your
Atlas Device SDK development. In React Native, use our @realm/react
hooks to manage the Atlas connection, authenticate users, and write to
the database. In Swift, use property wrappers and convenience
features designed to make it easier to work with SwiftUI.
struct SearchableDogsView: View { Dog.self) var dogs ( private var searchFilter = "" var body: some View { NavigationView { // The list shows the dogs in the realm. List { ForEach(dogs) { dog in DogRow(dog: dog) } } .searchable(text: $searchFilter, collection: $dogs, keyPath: \.name) { ForEach(dogs) { dogsFiltered in Text(dogsFiltered.name).searchCompletion(dogsFiltered.name) } } } } }
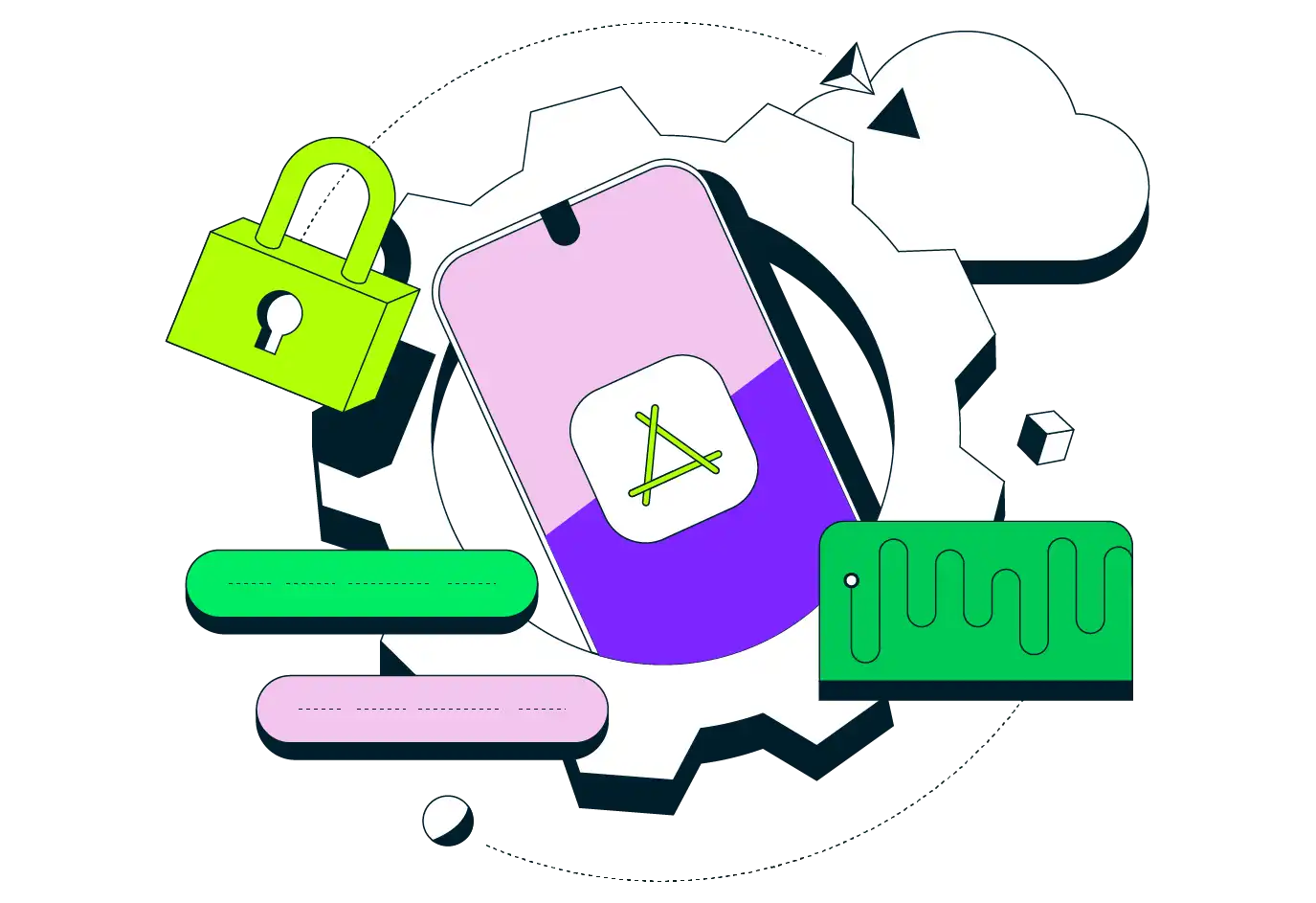